The buzz around application programming interfaces (APIs) is widespread, and for good reason. APIs provide a software interface that simplifies communication between two applications, a crucial aspect for achieving scalability and reusability. It’s increasingly common for proprietary web applications or services to include public APIs, allowing other developers to efficiently integrate features such as social media logins, credit card processing, and performance tracking.
In this blog, we will walk you through a thorough, step-by-step process of creating an Node.js API from scratch.
How to Create an API Using Node.js?
Node.js, a widely used JavaScript runtime powered by Chrome’s V8 JavaScript engine, has emerged as a prominent platform for creating server-side applications and APIs. APIs, which stand for Application Programming Interfaces, act as bridges that enable different software systems to communicate and interact with each other.
What is Rest API?
A REST (Representational State Transfer) API, commonly known as a RESTful API, is an architectural style used in the design of networked applications. It encompasses a set of principles and restrictions that dictate how resources are defined, structured, and accessed on the Internet.
In a REST API, resources are the central components that are made accessible and manipulated. A URL (Uniform Resource Locator) or URI (Uniform Resource Identifier) serves as a unique identifier for each resource. These resources can represent entities like users, products, posts, or any other type of data.
REST APIs are built upon a client-server model, where the server communicates with the client (for example, a web browser or a mobile app) by employing standardized HTTP methods such as GET, POST, PUT, DELETE, and more. These methods play a pivotal role in carrying out operations on the available resources, enabling tasks like retrieving data, creating new resources, updating existing ones, and deleting resources.
Setting Up the Development Environment for Node.js
1. Installing Node.js and npm
Installing Node.js and npm is the initial step in creating a Node.js API. Node.js serves as a JavaScript runtime for executing server-side JavaScript code, while npm, or Node Package Manager, streamlines the process of installing and managing Node.js packages.
To install Node.js, you should start by visiting the official Node.js website and obtaining the installer appropriate for your operating system. After the download, run the installer and carefully follow the on-screen instructions.
Once the installation is finished, you can verify whether Node.js and npm have been successfully installed on your system. You can do this by opening a terminal or command prompt and running the commands node -v and npm -v. If you see the versions of Node.js and npm displayed, it confirms the successful installation.
2. Initializing a Node.js Project
After installing Node.js and npm, you can initialize a Node.js project by following these steps:
1. Go to the specific directory in your terminal or command prompt where you plan to create your project.
2. Run the command “npm init” to initialize your project.
3. This command will interactively request project information like the package name, version, description, entry point, and more.
4. You can either input the required details or simply press Enter to accept the default values.
5. Once you’ve provided the necessary details or accepted the defaults, a file named “package.json” will be generated in your project directory.
6. The “package.json” file functions as a repository for metadata about your project and also compiles a list of the project’s dependencies.
By following these steps, you can easily establish a Node.js project and manage its configuration through the “package.json” file.
3. Installing Express.js Framework
Express.js, a popular web application framework for Node.js, simplifies the process of creating APIs and web applications. To add Express.js to your project, simply run the command npm install express in your project directory.
This command fetches the Express.js package from the npm registry and automatically adds it as a dependency in your package.json file. Additionally, it creates a node_modules directory in your project folder to house the installed packages. By following these steps, you can seamlessly incorporate Express.js and improve your web development workflow.
Now that you’ve successfully installed Express.js, you can begin harnessing its robust features and capabilities to construct your API. To integrate Express.js into your JavaScript files, simply utilize the ‘require’ keyword. From there, you can proceed to create routes, handle requests, and implement various middleware functions to enhance the functionality and performance of your API.
By establishing your development environment correctly, you’ve established the groundwork for building your Node.js API. The installation of Node.js and npm ensures you have the essential runtime and package management tools, and initializing a Node.js project and adding Express.js provides the structure and framework necessary for efficiently developing your API.
Steps to Create an API with Node.js
Here are the steps to build an API with Node.js:
Step 1: Project Setup
- Start by creating a fresh directory dedicated to your project.
- Now, open your terminal and go to the project directory.
- Kickstart a new Node.js project with the following command: npm init
Step 2: Install dependencies
You can install the necessary dependencies by executing the following command: Use the command npm install express body-parser to install the required package
Step 3: Create the server file
- Generate a new file within the project directory, for instance, name it server.js.
- At the beginning of the file, import the necessary modules by including them.

Create an instance of the Express application:

Add middleware to parse incoming request bodies:

Define the routes for your API.
- Start the server and listen on a specified port:
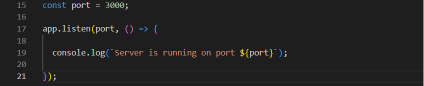
Step 4: Run the server
- Open the terminal and navigate to the project directory.
- Initiate the server by running the command: node server.js.
That’s all there is to it! You’ve now created a fundamental REST API with Node.js through Express. You can further enhance your API by including additional routes, connecting with databases, implementing authentication, and managing request payloads based on your requirements.
Conclusion
Creating a Node.js API involves several key steps, such as establishing endpoints, utilizing Node.js and Express, performing thorough testing, and deploying to a server or cloud environment. By implementing continuous integration and continuous deployment (CI/CD), you can optimize the development process. This method assures the creation of dependable and effective Node.js APIs that meet user needs and contribute to the growth of your business.